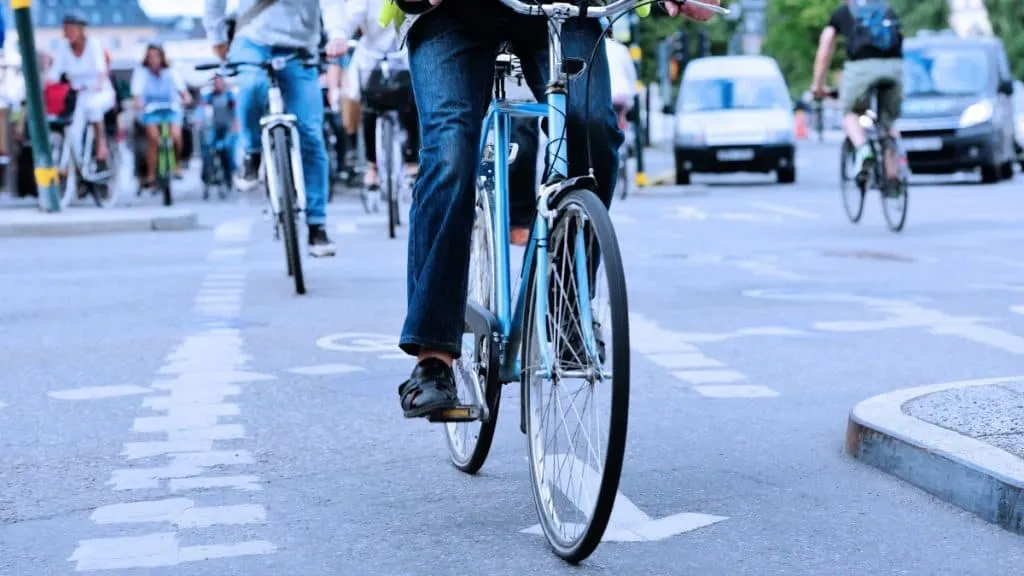
If you’re anything like me, once you fall in love with cycling, you want everybody to start enjoying the benefits it brings. Including your work colleagues. Commuting by bike is an excellent way to start your day, an to get others just as excited as you are, you need to know how to promote cycling.
This article contains 20 proven ways to promote cycling to work that your colleagues will not be able to resist. Read on and transform your work atmosphere into one you cannot wait to get to.
Be Clear that Cycling is Encouraged
Some people worry that not using a car may be seen as a disadvantage in the workplace. In America especially cycling is relatively new in terms of popularity, and there remains some uncertainty surrounding how employers are likely to react to their workers cycling to work.
Therefore one of the biggest ways to promote cycling is to simply encourage it! Make sure that your workers know that cycling is not only tolerated but applauded. You can make this clear with a simple email or announcement. If you have any employees that are already cycling to work, you can commend them in front of others.
Many of the following tips can give you a way to promote cycling on your own. Simply make sure to be enthusiastic about the measures you take so that your employees always know that your workplace is all for cycling!
Explain the Benefits of Cycling
There is no denying that people have become much more health-conscious in recent years. So, you may be able to get workers cycling to work by simply letting them know about what wonderful benefits cycling has.
Cycling to work will improve your overall health, allow you to avoid a myriad of germs on public transportation, and keep you away from traffic. People who exercise feel better and happier. In short cycling to work is both great for your health, your mood, and gives you far more control over your morning commute.
Making your employees aware of these benefits with even a simple handout may encourage those on the fence to give cycling a try. There are a ton of benefits from cycling that your workers should be made aware of including:
- Saving time
- Saving money
- More environmentally friendly
- Better health (both physical and mental)
- Avoiding traffic
- Better sleep
- Less exposure to germs
For more detail on the specific benefits that cycling brings, check out my article “23 Advantages of Cycling to Work”.
Create Safe Route Maps
Many people worry about cycling to work in traffic. Being on a bike on a busy roadway can be scary and dangerous. You can put many workers’ minds at ease by researching optimal biking routes to your place of work.
Back alleys and sideroads can all provide a more efficient and safer avenue for someone on a bike. Offering maps that highlight great bike-friendly approaches to your workplace could give many employees the information they need to overcome their initial fear about cycling to work.
People may also worry about the time it will take to cycle to work. Your route maps can also show average times for faster routes. Giving workers an idea of both which way to go and how long to plan can make starting cycling much less overwhelming!
Check Into Monetary Incentives
A bike may not be as expensive as a car, but the cost of a quality bike is still enough to make many workers hesitate. Biking will save money in the long run, but how do you get workers to take that initial fiscal plunge?
Offering some monetary encouragement may be the push your workers need to join the cycling craze. Whether it be upfront cash incentives or a program through which the company buys a bike and then takes a portion of the worker’s salary for several months to pay, money is a proven way to motivate employees.
You can also check out my article “How Much Money Can you Save By Cycling to Work?” to get some cool information to shout about at your work place!
Start a Biking Group
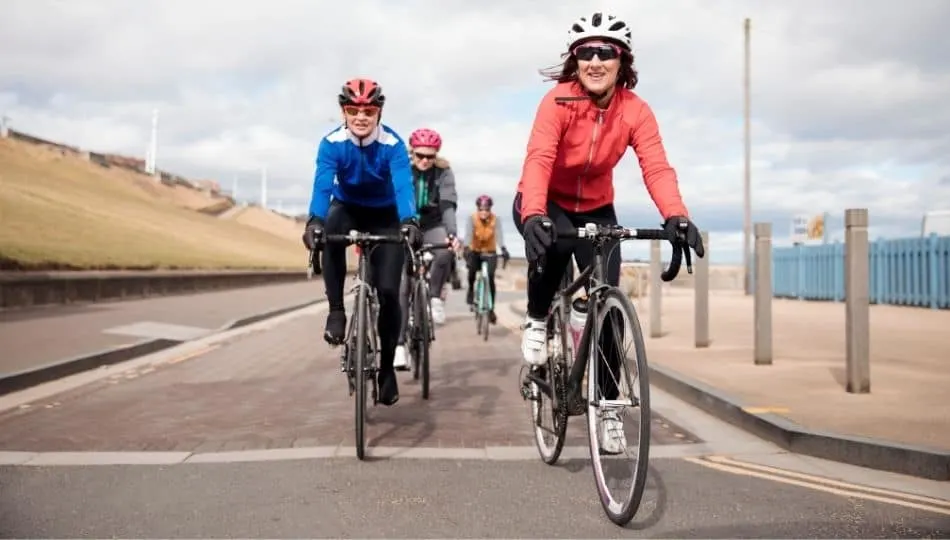
Whether we want to admit it or not, most of us feel much more comfortable doing something when we know we are not the only ones. People are much more likely to cycle to work if there is a cycling community at work for them to join. (It’s how I got started, and I haven’t looked back!)
There are a variety of apps you can use to get all of the cyclers at your work connected. With a community, cyclers can easily share routes, compete, ride together, and exchange other advice and tips.
Creating a community of bike riders will give people the support they need to get started and remain interested in cycling to work. Simply letting people know that they are not alone is sometimes the absolute best way to get people to invest in a new habit or idea.
It is through this group that you can also easily use many of the other tips on this list such as competitions, goals, and safe route maps. A group will not only encourage your workers to cycle, but it will make it much easier for you to promote cycling by keeping things in one place.
Secure Bike Parking
If you really want to get your workers to cycle to work regularly, you have to make it as easy as possible. A daily habit has to possess convenience for it to truly stick. If you want to encourage cycling to work, you have to provide facilities that will work for cyclists.
One of the most important things that cyclists need is a secure place to park their bikes. A quality bike costs quite a lot of money, and your workers need to feel that their investment will be protected if they choose to cycle to work.
Secure bike parking should be both in a covered area and have security. If your company already has a parking deck, you can easily install bike racks. If your company does not have the space or funds for bike racks, then allowing employees to bring their bikes into the building could be a potential solution.
Your bike parking could also include a few essential items like an air pump and flat tire kit. Making sure your employees have what they need to succeed is essential for promoting any new idea. Whatever the exact solution, workers must have a safe place to put their bikes if you want them to cycle to work.
Ensure a Comfortable Space to Change
Another important facility to provide for cyclists in your workplace is a place to change and wash. Cycling is exercise after all and thus can result in some sweaty employees. In addition cycling in office wear is just plain uncomfortable. To get workers to cycle to work, they need to be able to do so comfortably, which is why providing a changing area is so important.
If you give employees a place to change, they will be far less likely to try cycling to work in their office clothes. Workers who try to cycle in work clothes will quickly become uncomfortable and may give up cycling to work altogether. Give your workers a place to change and encourage them to use it.
The best changing area would be one with both showers and lockers. This would allow workers both to wash off the sweat of a ride and to store their cycling clothes and gear safely. No one wants to work while they are sweaty! Providing showers will get your employees to cycle and make them more productive by helping them start the day feeling refreshed.
If you cannot yet provide showers, offering storage for cycling gear, a bathroom to change, and a large sink for washing up can be a great way to gauge interest. If the number of cyclists increases, that is a sign that a showering facility is probably worth the investment.
Try a Trial Day
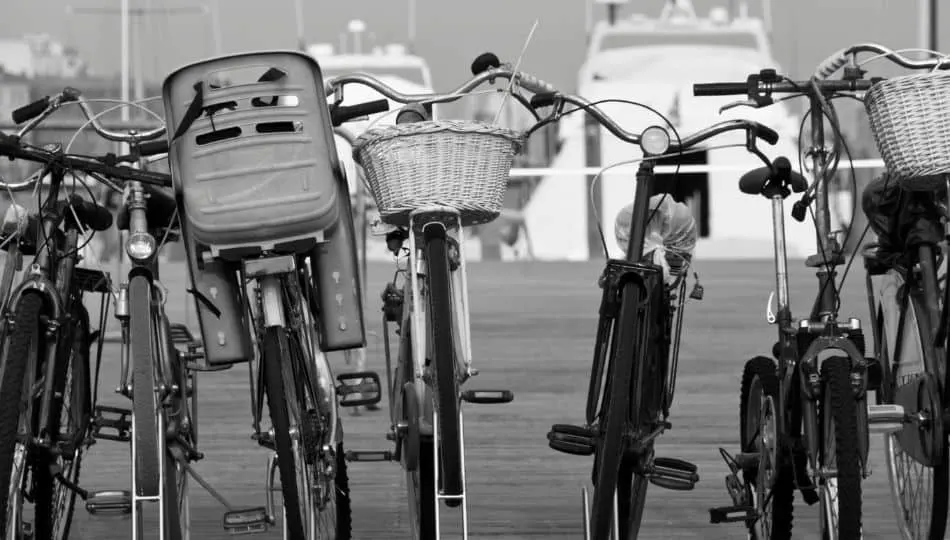
Cycling is a commitment, and buying a good bike is an investment. You may have workers who are interested in cycling, but reluctant to invest in something they have never tried. To promote interest and give workers a chance to see if cycling for them having a Cycle to Work Day is a great idea!
A Cycle to Work Day gives employees a chance to test out cycling with no risk to them. They can borrow a bike for a one-day test trial, which will give them a chance to check the distance, traffic, and more before putting money down.
A Cycle to Work Day is also a clear way to say that an employer encourages cycling. Such days serve the double purpose of promotion and test trials to see how well cycling will work for your employees.
Friendly Competition
Another way to encourage cycling is to create workplace competitions. A little friendly competition can go a long way in motivating people to get on their bikes!
Competitions can address a variety of different components such as number of miles biked, average speed, number of days cycled to work, and more. Offering small prizes with these competitions is another great way to make clear your support for cycling.
Friendly competitions also serve to strengthen the cycling community in your workplace. Having a community is key to creating a sustained cycling group, and competitions are an excellent way to do that.
To get people invested, your work could even hold races or a competition day where everyone can show off their cycling skills. Make cycling something to be proud of, and more people will be bound to participate.
Offer Bicycle Training
Some people may be interested in cycling but have no idea how to get started. Offering some basic bike training can give workers the confidence to give cycling to work a try.
There are several different ways to offer cycling training to employees. A weekend workshop could be a great way to handle a large interest group. You could also compile a list of online resources such as videos to send to any interested employees.
Many people think of cycling as the same as riding a bike when they were a kid. While cycling is certainly accessible, your employees might run into problems before they truly get started without the proper training.
A training program can teach your workers how to signal when biking on roadways, the proper stance for riding to avoid aches and pains, how to care for your bike, and how to change a flat tire. Giving your employees the knowledge to be successful with their cycling will go a long way towards creating consistently cycling workers.
A Bike Share Program
Investing money in an activity that they have not fully tried is a source of reluctance for many people. How do you convince people that never bike that they should start cycling to work every day? It can help to let your workers become more familiar with biking before taking the leap to cycling to work.
A bike-share program is a great way to give your employees a taste of biking. Investing in a few company bikes can allow your employees to test out biking while running company errands, grabbing lunch, or just getting some exercise on breaks. Many cities also now have bike-share programs, which your business can utilize, so you may not need to buy company bikes.
Bike-sharing allows workers to become familiar with biking so that they experience both how enjoyable and easy cycling is. Essentially bike-sharing offers a taste that will encourage your workers to take the full plunge and buy a bike themselves.
Encourage Workers to Purchase a Quality Bike
Just as giving workers some basic cycling training can help them get started and stick with the habit, advising about the actual purchasing of a bike may also prove useful for creating a group of cycling workers.
Your workers may be tempted to purchase a $100 bike from a local Walmart, but in general, this is usually a bad idea. While they may initially balk at the extra price, your workers must understand that they should invest in a quality bike for commuting purposes.
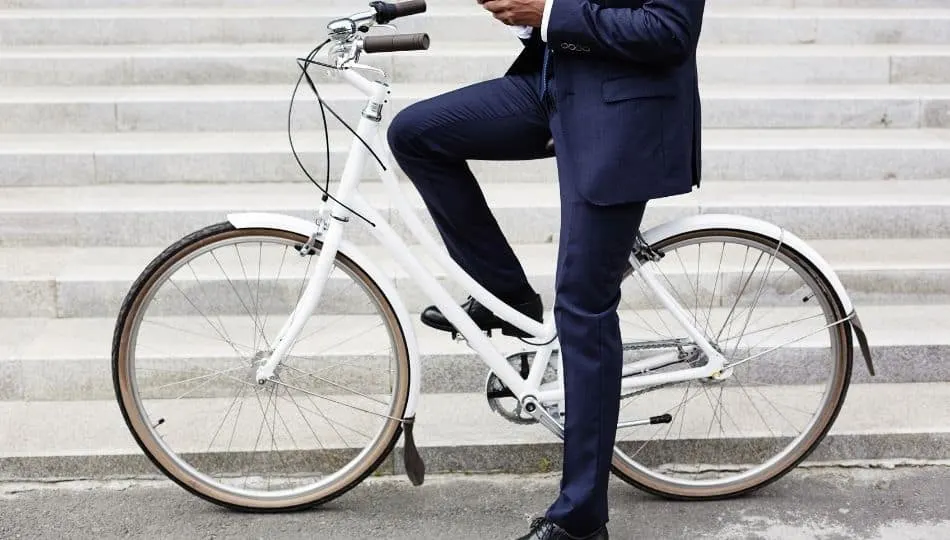
If they are going to ride a bike every day, it has to be comfortable, light, and durable. This bike is going to get them to and from work, and it needs to be high quality to ensure that it is safe on roadways and provides the best experience. Workers will quickly stop cycling to work if their bike is uncomfortable or broken.
A good bike should cost a worker several hundred dollars. If you can get your workers to start out with good gear, they are much more likely to enjoy and continue their cycling.
Connect with a Local Bike Shop
No matter how invested you and your workplace may be in cycling, you will never know as much as someone whose livelihood depends on bikes and cycling. One of the best ways to locate the resources and advice workers need to be successful with cycling is to connect with a local bike shop.
Finding a trusted local shop to recommend to employees and maybe even partner with for training and competitions has several advantages. Knowing where to purchase a bike and gear can smooth over the process of getting started with cycling for many. A bike shop can help you with cycling information that your workers might enjoy or need to know.
Whatever you do not know about cycling can be filled in with the help of a bike shop. You and your employees can feel better knowing that you know experts which can answer questions and make entering the world of cycling easier.
Create Bikepools
You have heard about carpooling, so what about the same thing with bikes? For those new to cycling, doing it in a group can reduce a lot of the potential worry and stress. Encourage your work’s cyclists to share their routes. If there is overlap plan a day for the more experienced rider to cycle to work along with those newer to cycling.
A group commute could get those worried about navigating and traffic to give cycling a try. It may also make some workers feel more comfortable in terms of general safety. Not to mention social biking is way more fun! If you create a positive experience while biking, workers will be far more inclined to try to continue the habit.
Make It Part of a Larger Green Initiative
In the 21st century, humans have become astronomically more aware of our impact on the environment. Many of us, both individuals and companies, are trying to do better, and cycling to work is a natural fit for such goals.
If your work is already taking steps to be greener with other things such as recycling, energy efficiency, and lower waste, then suggesting a cycle to work initiative makes perfect sense! Placing cycling to work within a larger green initiative may allow you to use already existing resources to make cycling to work easier and more accessible with some of our other tips.
Plus, if your workers are already dedicated to a green initiative it may be easier to convince them to give cycling a try. If they care enough to take some steps, it is a good sign that they might be open to trying cycling!
Start a Fitness Challenge
While competitions specifically related to biking may be a bit more straightforward, initiating a fitness challenge at work can also serve to get people cycling. Using Fitbits, Apple watches, or other tracking devices, workers can compete with each other in various fitness challenges. Your entire workplace can even compete against a rival company to see who has the fittest employees.
Simply encouraging your employees to be more fit, in general, is a great way to get them to dust off old bikes. You can even suggest cycling to work as a way to work on the fitness challenge without cutting into their free time.
Create a Company Cycling Goal
Unfortunately, as with many good things, the benefits of cycling make not appear immediately for many. It takes a bit of commitment before people start to notice improved health and the time and money they are saving. Offering some form of short-term gratification can help your workers get over the bump to see the long-term benefits of cycling to work.
One way to do this is with a company-wide cycling goal. Something as simple as offering a free lunch for those who cycle to work for 5 days in a row, or a small bonus when you reach a certain number of employees cycling to work can be a great way to provide that little push many need to start a new habit.
Give Away a Bike
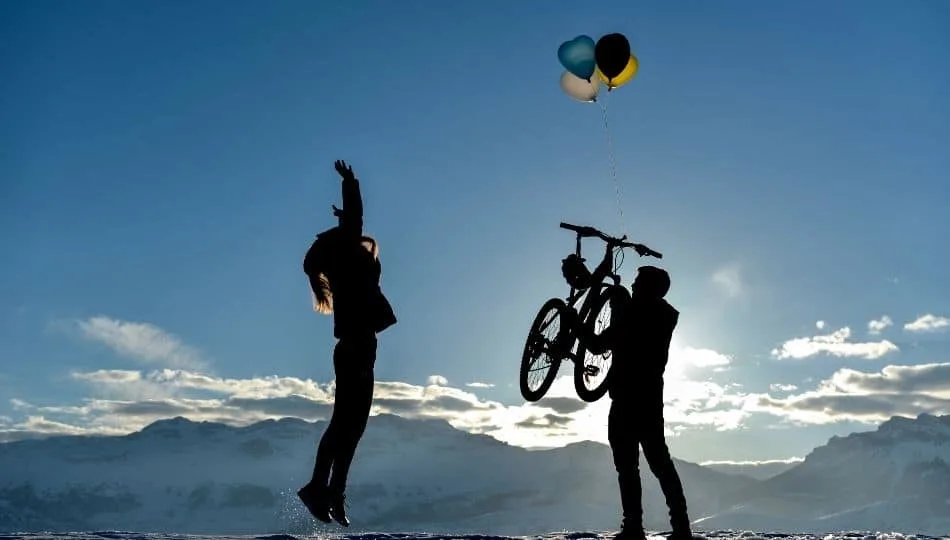
Your company probably lacks the funds to give a bike to every single employee, but one great promotional idea is to give one employee a bike. A raffle giving away a bike sends a clear message to employees about how the company values cycling.
You can turn a bike raffle into a large event by spending a week building up excitement before the drawing. This is a great time to inform your employees about the benefits of biking and to present them with route maps and other resources.
After giving away the bike, you can offer monetary incentives or a monthly payment program for other interested workers. Something as simple as a raffle is a great way to build momentum around an idea which you can then use to propel forward with many of the other tips in this article.
Talk About Alternatives to Normal Bikes
Some people may feel awkward about cycling to work on a normal bike for many reasons. Whether it be their fitness level, a bad biking experience, or a longer commute, some people do not like the idea of regular cycling to work, but that does not mean you should give up on them.
Electric and hybrid bikes can be a great alternative for those who have a harder time cycling or who have a lengthy commute. After all pedaling tow miles is quite different that pedaling seven miles! Simply making your employees aware of other options could provide an option for those for whom normal cycling is not a realistic option.
There are other forms of non-automotive transportation as well. Electric scooters are also an option. Being open to methods besides the traditional bike will ensure that your workers can choose whatever method is most accessible to them. Promoting cycling to work should never turn into shaming employees but should seek to find what works best for workers.
Do It Yourself
You should never expect your workers to do something you are unwilling to do yourself. All of the encouragement and promotions in the world will do little good if you show yourself unwilling to try cycling yourself.
Good leaders lead by example. Showing up to work on your own bike will send a clearer message than just about anything else you can do to promote cycling. There is no better way to say clearly and loudly that a company wants its workers to try cycling to work than having the boss show up on a bike.